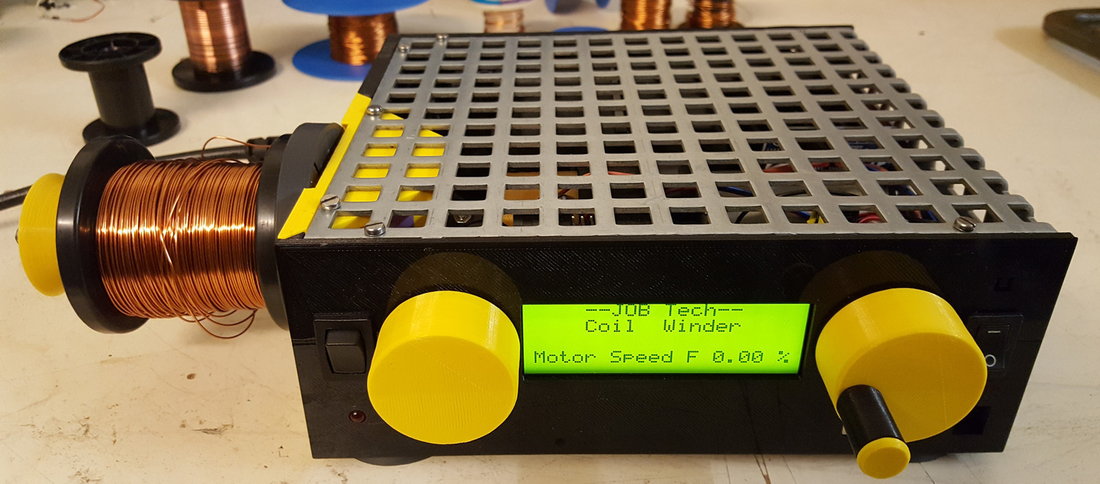
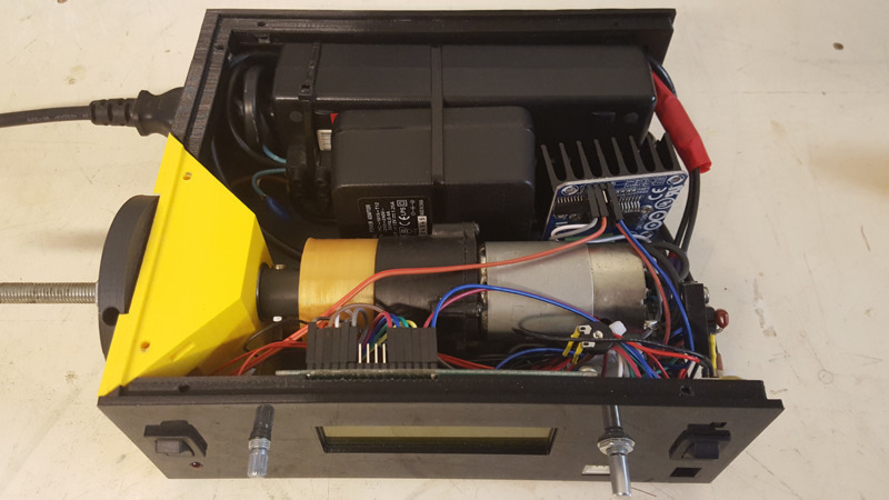
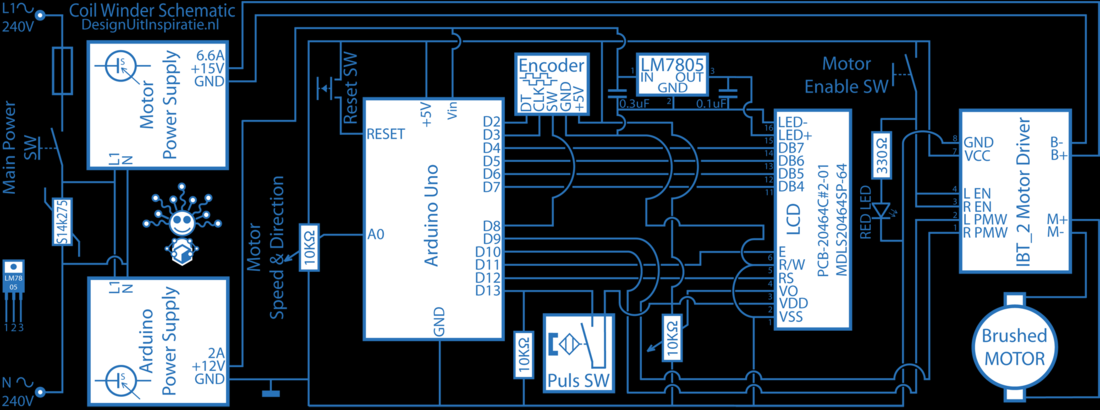
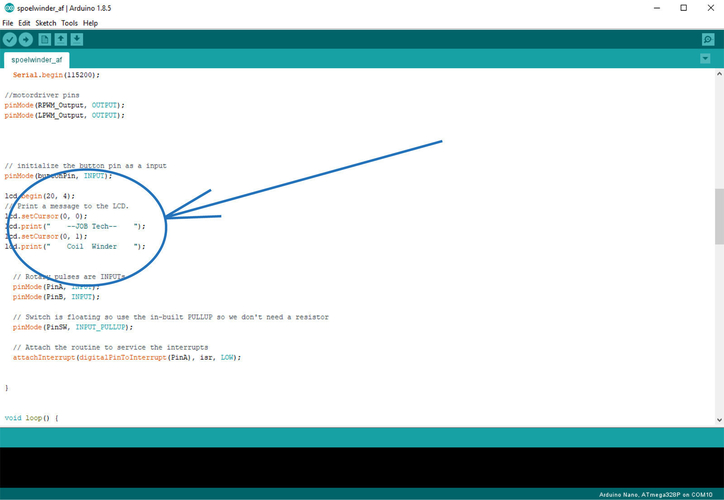
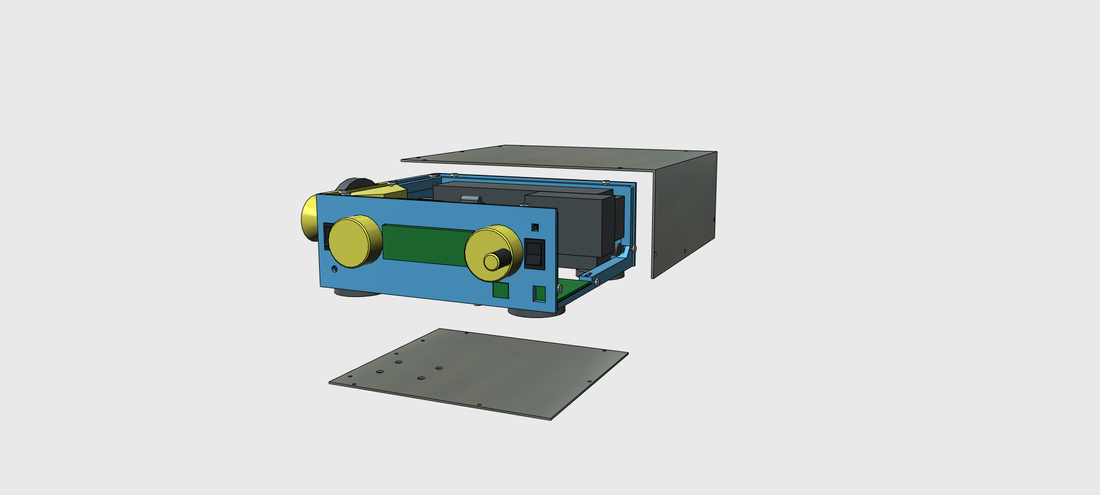
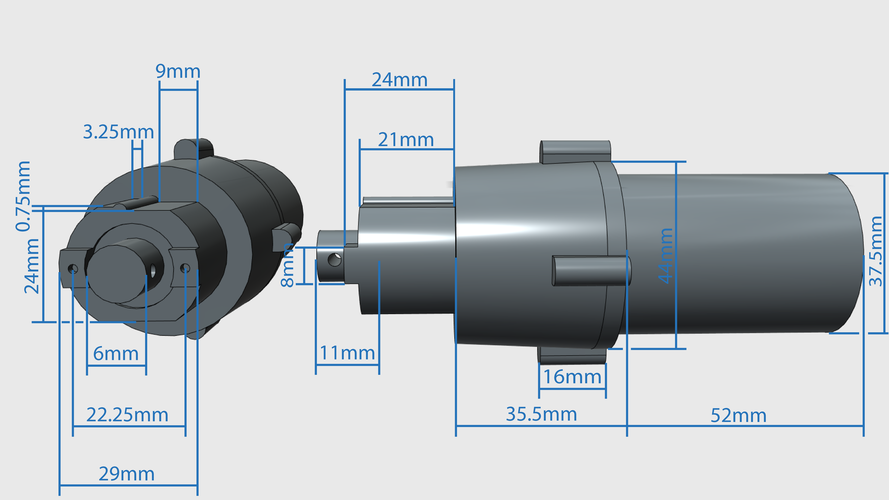
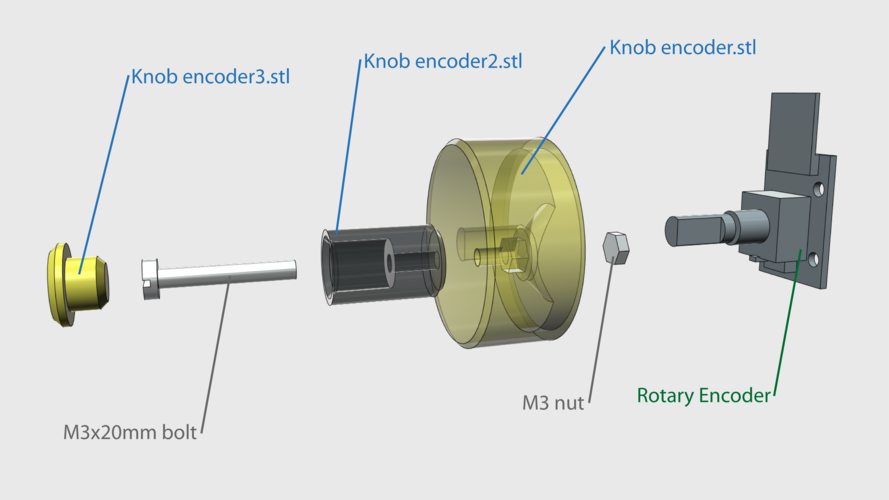
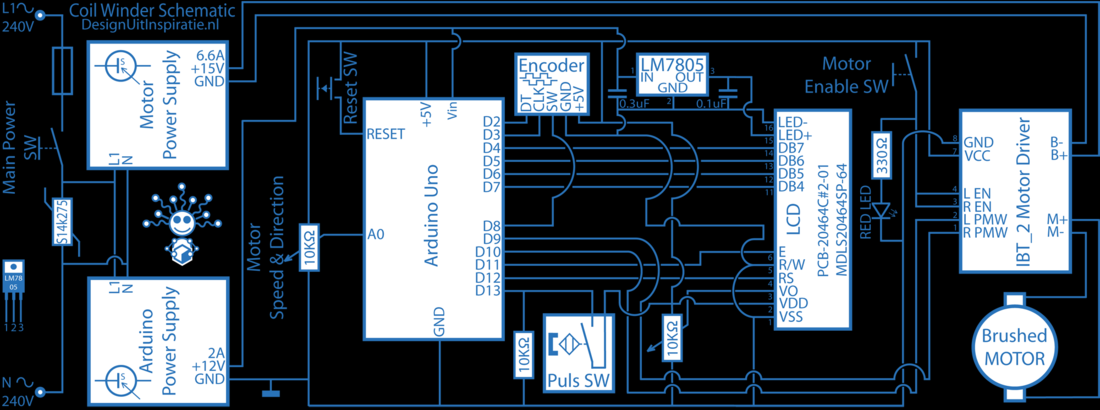
Prints (0)
-
No Prints Yet
Be the first to upload a Print for this Design!
Description
This is a simple machine to make Coils. It has it's own internal counter, that counts the winding's that are made. You can set an amount of winding's in advance. The machine will stop automatically, when the set amount of winding's is reached.
sketch--
// include the library #include <LiquidCrystal.h>
// this constant won't change const int buttonPin = 13; // the pin that the pushbutton is attached to //const int ledPin = 13; // the pin that the LED is attached to
// initialize the library by associating any needed LCD interface pin // with the arduino pin number it is connected to const int rs = 12, en = 11, d4 = 7, d5 = 6, d6 = 5, d7 = 4; LiquidCrystal lcd(rs, en, d4, d5, d6, d7);
// Variables will change int buttonPushCounter = 0; // counter for the number of button presses int buttonState = 0; // current state of the button int lastButtonState = 0; // previous state of the button
//for motor driver and pot regulation of speed int SENSOR_PIN = A0; // center pin of the potentiometer int RPWM_Output = 9; // Arduino PWM output pin 5; connect to IBT-2 pin 1 (RPWM) int LPWM_Output = 10; // Arduino PWM output pin 6; connect to IBT-2 pin 2 (LPWM)
// Used for generating interrupts using CLK signal const int PinA = 2;
// Used for reading DT signal const int PinB = 3;
// Used for the push button switch const int PinSW = 8;
// Keep track of last rotary value int lastCount = 0;
//save stat of virtualposition int saveState =1;
// Updated by the ISR (Interrupt Service Routine) volatile int virtualPosition = 0;
void isr () { static unsigned long lastInterruptTime = 0; unsigned long interruptTime = millis();
// If interrupts come faster than 5ms, assume it's a bounce and ignore if (interruptTime - lastInterruptTime > 5) { if (digitalRead(PinB) == LOW) { virtualPosition--; // Could be -5 or -10 } else { virtualPosition++; // Could be +5 or +10 }
// Restrict value so the selected windings option cannot go below 0 virtualPosition = min(10000, max(0, virtualPosition));
} // Keep track of when we were here last (no more than every 5ms) lastInterruptTime = interruptTime; }
void setup() { // view output on serial monitor Serial.begin(115200);
//motordriver pins pinMode(RPWM_Output, OUTPUT); pinMode(LPWM_Output, OUTPUT);
// initialize the button pin as a input pinMode(buttonPin, INPUT);
lcd.begin(20, 4); // Print a message to the LCD. lcd.setCursor(0, 0); lcd.print(" --JOB Tech-- "); lcd.setCursor(0, 1); lcd.print(" Coil Winder ");
// Rotary pulses are INPUTs pinMode(PinA, INPUT); pinMode(PinB, INPUT);
// Switch is floating so use the in-built PULLUP so we don't need a resistor pinMode(PinSW, INPUT_PULLUP);
// Attach the routine to service the interrupts attachInterrupt(digitalPinToInterrupt(PinA), isr, LOW);
}
void loop() { int sensorValue = analogRead(SENSOR_PIN);
int Stop = (saveState); label: if (saveState < buttonPushCounter+1) { analogWrite(LPWM_Output, 0); analogWrite(RPWM_Output, 0); lcd.setCursor(0, 1); lcd.print ("READY!!! :-)"); lcd.setCursor(0, 2); lcd.print ("----Please Reset----"); goto label; } // sensor value is in the range 0 to 1023 // the lower half of it we use for reverse rotation; the upper half for forward rotation if (sensorValue < 512) { // reverse rotation int reversePWM = -(sensorValue - 511) / 2; analogWrite(LPWM_Output, 0); analogWrite(RPWM_Output, reversePWM); float motorSpeedR = reversePWM * (200.0 / 511.0); lcd.setCursor(0, 3); lcd.print ("Motor Speed R "); lcd.print (motorSpeedR); lcd.setCursor(19, 3); lcd.print ("%"); // Serial.println (" Speed Reverse"); // Serial.print (motorSpeedR); //Serial.print ("%"); } else { // forward rotation int forwardPWM = (sensorValue - 512) / 2; analogWrite(LPWM_Output, forwardPWM); analogWrite(RPWM_Output, 0);
float motorSpeedL = forwardPWM * (200.0 / 511.0); lcd.setCursor(0, 3); lcd.print ("Motor Speed F "); lcd.print (motorSpeedL); lcd.setCursor(19, 3); lcd.print ("%"); }
// read the pushbutton input pin: buttonState = digitalRead(buttonPin);
// compare the buttonState to its previous state if (buttonState != lastButtonState) { // if the state has changed, increment the counter if (buttonState == HIGH) { // if the current state is HIGH then the button went from off to on buttonPushCounter++; //print counted button presses on LCD lcd.setCursor(0, 0); lcd.print("Windings Done "); lcd.print(buttonPushCounter); } // Delay a little bit to avoid bouncing delay(50); } // save the current state as the last state, for next time through the loop lastButtonState = buttonState;
// Is someone pressing the rotary switch? if ((!digitalRead(PinSW))) { saveState = virtualPosition; while (!digitalRead(PinSW)) delay(10); //print the set amount of windings on the LCD lcd.setCursor(0, 2); lcd.print("Amount Set "); lcd.print(saveState); }
// If the current rotary switch position has changed then update everything if (virtualPosition != lastCount) {
//print the selected amount of windings on the LCD lcd.setCursor(0, 1); lcd.print("Select Amount "); lcd.print(virtualPosition);
// Keep track of this new value lastCount = virtualPosition ; }
}
Comments